Day 1 - Lecture 2
Contents
2. Day 1 - Lecture 2#
2.1. Section 2. File handling and functions#
2.1.1. 1) Opening a text file#
# To open a text based file, we can use the open() function.
f_obj = open('../data/file_example1.txt')
# Let's print f_obj to see what it is.
print(f_obj)
<_io.TextIOWrapper name='../data/file_example1.txt' mode='r' encoding='UTF-8'>
# We can see the entire text by using the read() function.
#print(f_obj.read())
# Or we can print the text by lines using the readline() function.
print(f_obj.readline())
print(f_obj.readline())
xvals yvals
0.8641216311612758 0.23919054492654035
# We can also iterate through the file object.
for i in f_obj:
print(i)
break # break here to avoid printing the entire file.
# As you can see, the f_obj.readline() moved the file-pointer. Let's reset it to the beginning of the line and extract xvals and yvals.
f_obj.seek(0)
xvals = []
yvals = []
f_obj.readline() # To remove the column names
for line in f_obj:
x, y = line.split()
xvals.append(float(x))
yvals.append(float(y))
import matplotlib.pyplot as plt
plt.scatter(xvals, yvals)
plt.show()
0.2131415384891292 0.9434728716309931
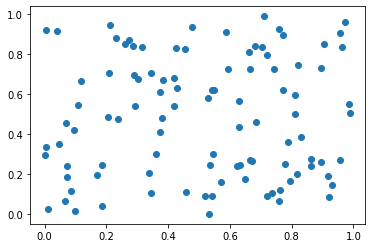
# We will .
xvals_new = xvals[0::2]
yvals_new = yvals[0::2]
# To test the indexing.
#test_list = list(range(100))
#print(test_list[0::2])
plt.scatter(xvals_new, yvals_new)
print(len(xvals_new), len(yvals_new))
# Writing the x and y values
with open('../data/file_example1_after.txt', 'w') as f_obj:
# Column names
f_obj.write('xvals yvals \n')
for x, y in zip(xvals, yvals):
# Forming a line of string
new_line = str(x)+ ' ' +str(y) + '\n'
f_obj.write(new_line)
50 50
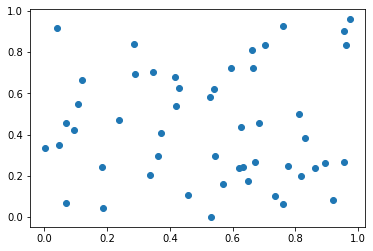
2.1.2. 2) Counting words from a text file#
# We will be using the file_example2.txt to count the each words.
# Define an empty dictionary
word_dict = {}
# Things need to be removed
removing_list = ['.', '-', ',', '!', '?', ':', ';']
# Opening a file
with open('../data/file_example2.txt') as f_obj:
for line in f_obj:
# Splitting a line of string into words
splitted = line.split()
for word in splitted:
# Remove leading and trailing whitespaces
word = word.strip()
# Force the words to be lowercase
word = word.lower()
# Remove punctuation marks
for remove in removing_list:
while remove in word:
word = word.replace(remove,'')
# Save it in the dictionary
if word in word_dict.keys():
word_dict[word] += 1
else:
word_dict[word] = 1
print(word_dict)
# Can you write a file with two columns named "Words" and "Counts?"
{'call': 1, 'me': 6, 'ishmael': 1, 'some': 8, 'years': 1, 'ago': 1, '': 13, 'never': 1, 'mind': 1, 'how': 2, 'long': 1, 'precisely': 1, 'having': 1, 'little': 2, 'or': 4, 'no': 2, 'money': 2, 'in': 23, 'my': 4, 'purse': 1, 'and': 31, 'nothing': 3, 'particular': 1, 'to': 21, 'interest': 1, 'on': 3, 'shore': 1, 'i': 9, 'thought': 1, 'would': 2, 'sail': 1, 'about': 2, 'a': 25, 'see': 4, 'the': 52, 'watery': 1, 'part': 1, 'of': 35, 'world': 1, 'it': 13, 'is': 17, 'way': 2, 'have': 1, 'driving': 1, 'off': 2, 'spleen': 1, 'regulating': 1, 'circulation': 1, 'whenever': 4, 'find': 2, 'myself': 2, 'growing': 1, 'grim': 1, 'mouth': 1, 'damp': 1, 'drizzly': 1, 'november': 1, 'soul': 2, 'involuntarily': 1, 'pausing': 1, 'before': 2, 'coffin': 1, 'warehouses': 2, 'bringing': 1, 'up': 3, 'rear': 1, 'every': 3, 'funeral': 1, 'meet': 1, 'especially': 1, 'hypos': 1, 'get': 4, 'such': 2, 'an': 2, 'upper': 1, 'hand': 1, 'that': 7, 'requires': 1, 'strong': 1, 'moral': 1, 'principle': 1, 'prevent': 1, 'from': 6, 'deliberately': 1, 'stepping': 1, 'into': 3, 'street': 1, 'methodically': 1, 'knocking': 1, 'people’s': 1, 'hats': 1, 'then': 2, 'account': 1, 'high': 3, 'time': 3, 'sea': 3, 'as': 9, 'soon': 1, 'can': 2, 'this': 8, 'substitute': 1, 'for': 5, 'pistol': 1, 'ball': 1, 'with': 6, 'philosophical': 1, 'flourish': 1, 'cato': 1, 'throws': 1, 'himself': 1, 'upon': 7, 'his': 8, 'sword': 1, 'quietly': 1, 'take': 3, 'ship': 2, 'there': 11, 'surprising': 1, 'if': 5, 'they': 7, 'but': 8, 'knew': 1, 'almost': 3, 'all': 12, 'men': 3, 'their': 2, 'degree': 1, 'other': 2, 'cherish': 1, 'very': 1, 'nearly': 1, 'same': 2, 'feelings': 1, 'towards': 1, 'ocean': 2, 'now': 2, 'your': 5, 'insular': 1, 'city': 2, 'manhattoes': 1, 'belted': 1, 'round': 1, 'by': 6, 'wharves': 1, 'indian': 1, 'isles': 1, 'coral': 1, 'reefs': 1, 'commerce': 1, 'surrounds': 1, 'her': 1, 'surf': 1, 'right': 1, 'left': 1, 'streets': 2, 'you': 13, 'waterward': 1, 'its': 2, 'extreme': 1, 'downtown': 1, 'battery': 1, 'where': 1, 'noble': 1, 'mole': 1, 'washed': 1, 'waves': 1, 'cooled': 1, 'breezes': 1, 'which': 2, 'few': 1, 'hours': 1, 'previous': 1, 'were': 6, 'out': 2, 'sight': 2, 'land': 4, 'look': 2, 'at': 2, 'crowds': 2, 'water': 8, 'gazers': 1, 'circumambulate': 1, 'dreamy': 1, 'sabbath': 1, 'afternoon': 1, 'go': 3, 'corlears': 1, 'hook': 1, 'coenties': 1, 'slip': 1, 'thence': 1, 'whitehall': 1, 'northward': 1, 'what': 4, 'do': 2, 'posted': 1, 'like': 2, 'silent': 1, 'sentinels': 1, 'around': 1, 'town': 1, 'stand': 4, 'thousands': 2, 'mortal': 1, 'fixed': 2, 'reveries': 2, 'leaning': 1, 'against': 1, 'spiles': 1, 'seated': 1, 'pierheads': 1, 'looking': 1, 'over': 1, 'bulwarks': 1, 'ships': 2, 'china': 1, 'aloft': 1, 'rigging': 1, 'striving': 1, 'still': 2, 'better': 1, 'seaward': 1, 'peep': 1, 'these': 1, 'are': 4, 'landsmen': 1, 'week': 1, 'days': 1, 'pent': 1, 'lath': 1, 'plaster': 1, 'tied': 1, 'counters': 1, 'nailed': 1, 'benches': 1, 'clinched': 1, 'desks': 1, 'green': 1, 'fields': 1, 'gone': 1, 'here': 5, 'come': 2, 'more': 2, 'pacing': 1, 'straight': 1, 'seemingly': 1, 'bound': 1, 'dive': 1, 'strange': 1, 'will': 3, 'content': 1, 'them': 3, 'extremest': 1, 'limit': 1, 'loitering': 1, 'under': 1, 'shady': 1, 'lee': 1, 'yonder': 2, 'not': 4, 'suffice': 1, 'must': 1, 'just': 1, 'nigh': 1, 'possibly': 1, 'without': 2, 'falling': 1, 'miles': 3, 'leagues': 1, 'inlanders': 1, 'lanes': 1, 'alleys': 1, 'avenues': 1, 'north': 1, 'east': 1, 'south': 1, 'west': 1, 'yet': 2, 'unite': 1, 'tell': 1, 'does': 1, 'magnetic': 1, 'virtue': 1, 'needles': 1, 'compasses': 1, 'those': 1, 'attract': 1, 'thither': 1, 'once': 1, 'say': 1, 'country': 1, 'lakes': 1, 'any': 1, 'path': 1, 'please': 1, 'ten': 1, 'one': 3, 'carries': 1, 'down': 2, 'dale': 1, 'leaves': 2, 'pool': 1, 'stream': 2, 'magic': 2, 'let': 1, 'most': 2, 'absentminded': 1, 'be': 4, 'plunged': 2, 'deepest': 1, 'man': 1, 'legs': 1, 'set': 1, 'feet': 1, 'agoing': 1, 'he': 6, 'infallibly': 1, 'lead': 1, 'region': 1, 'should': 1, 'ever': 2, 'athirst': 1, 'great': 1, 'american': 1, 'desert': 1, 'try': 1, 'experiment': 1, 'caravan': 1, 'happen': 1, 'supplied': 1, 'metaphysical': 1, 'professor': 1, 'yes': 1, 'knows': 1, 'meditation': 1, 'wedded': 1, 'artist': 1, 'desires': 1, 'paint': 1, 'dreamiest': 1, 'shadiest': 1, 'quietest': 1, 'enchanting': 1, 'bit': 1, 'romantic': 1, 'landscape': 1, 'valley': 1, 'saco': 1, 'chief': 1, 'element': 1, 'employs': 1, 'trees': 1, 'each': 1, 'hollow': 1, 'trunk': 1, 'hermit': 1, 'crucifix': 1, 'within': 1, 'sleeps': 1, 'meadow': 1, 'sleep': 1, 'cattle': 1, 'cottage': 1, 'goes': 1, 'sleepy': 1, 'smoke': 1, 'deep': 1, 'distant': 1, 'woodlands': 1, 'winds': 1, 'mazy': 1, 'reaching': 1, 'overlapping': 1, 'spurs': 1, 'mountains': 1, 'bathed': 1, 'hillside': 1, 'blue': 1, 'though': 2, 'picture': 1, 'lies': 1, 'thus': 1, 'tranced': 1, 'pinetree': 1, 'shakes': 1, 'sighs': 1, 'shepherd’s': 2, 'head': 1, 'vain': 1, 'unless': 1, 'eye': 1, 'him': 3, 'visit': 1, 'prairies': 1, 'june': 1, 'when': 2, 'scores': 2, 'wade': 1, 'kneedeep': 1, 'among': 1, 'tigerlilies': 1, 'charm': 1, 'wanting': 1, 'drop': 1, 'niagara': 1, 'cataract': 1, 'sand': 1, 'travel': 1, 'thousand': 1, 'why': 5, 'did': 4, 'poor': 1, 'poet': 1, 'tennessee': 1, 'suddenly': 1, 'receiving': 1, 'two': 1, 'handfuls': 1, 'silver': 1, 'deliberate': 1, 'whether': 1, 'buy': 1, 'coat': 1, 'sadly': 1, 'needed': 1, 'invest': 1, 'pedestrian': 1, 'trip': 1, 'rockaway': 1, 'beach': 1, 'robust': 2, 'healthy': 2, 'boy': 1, 'crazy': 1, 'first': 2, 'voyage': 1, 'passenger': 1, 'yourself': 1, 'feel': 1, 'mystical': 1, 'vibration': 1, 'told': 1, 'old': 1, 'persians': 1, 'hold': 1, 'holy': 1, 'greeks': 1, 'give': 1, 'separate': 1, 'deity': 1, 'own': 1, 'brother': 1, 'jove': 1, 'surely': 1, 'meaning': 2, 'deeper': 1, 'story': 1, 'narcissus': 1, 'who': 1, 'because': 1, 'could': 1, 'grasp': 1, 'tormenting': 1, 'mild': 1, 'image': 3, 'saw': 1, 'fountain': 1, 'was': 1, 'drowned': 1, 'we': 1, 'ourselves': 1, 'rivers': 1, 'oceans': 1, 'ungraspable': 1, 'phantom': 1, 'life': 1, 'key': 1}
2.1.3. 3) Functions#
We will define a simple function that can do simple math.
# Here is a function that can add two numbers
# To show that these variables outside of the function won't affect the variables inside.
a = 3
b = 5
def add(a, b):
result = a + b
return result
print(a + b)
print(add(1,2))
def calculator(a, operator, b):
operations = ['+', '-', '*', '/', '^']
if operator == '+':
result = a + b
elif operator == '-':
result = a - b
elif operator == '*':
result = a * b
elif operator == '/':
result = a / b
elif operator == '^':
result = a ** b
else:
raise RuntimeError('Check your operator')
return result
print("Testing the calculator function")
print(calculator(3,'+',5))
print(calculator(3,'-',5))
print(calculator(3,'*',5))
print(calculator(3,'^',5))
# To raise the error
#print(calculator(3,'&',5))
8
3
Testing the calculator function
8
-2
15
243
Now, we will define a function that can parse through a complicated text file.
# We will extract important information from a complicated text file.
# It is a quantum calculation output file from a software package.
def parse_output(input_name, output_name):
# Defining an empty list that will be returned
energies = []
# Open a file
with open(input_name) as f_obj:
# Iterate through the lines
for line in f_obj:
# If desired string is detected,
if 'FINAL ENERGY' in line:
# Save the number.
energies.append(float(line.split()[2]))
# Save the extracted values
with open(output_name, 'w') as f_obj:
# %12 holds 12 spaces for the 's' type (string) data
f_obj.write("%12s %12s \n" %("Iteration", "Energy"))
for i, energy in enumerate(energies):
line = '%12s %12.5f \n' %('Iteration %i' %i, energy)
f_obj.write(line)
return energies
energies = parse_output('../data/file_example3.out','../data/energies.txt')